IF then statement is used to run the certain code when given condition is true and if condition is false then some other code will execute. We must close the IF statement with End IF.
In the below example we are asking a question by using Message box. If user clicks on Yes, then we know it will return 6 and if user clicks on No then it will return 7. So, we have taken condition to show the message box for the click of yes and no.
Sub IF_else_Then() Dim s As Integer s = MsgBox("Do you like VBA?", vbQuestion + vbYesNo) If s = 6 Then MsgBox "You clicked on Yes" Else MsgBox "You clicked on No" End If End Sub
When we run this code, it will show below given message.
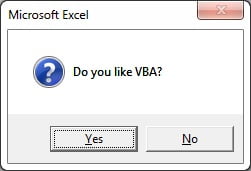
If you click on Yes button, then it will return-
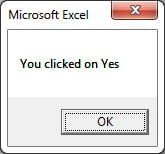
If you click on No button, then it will return-
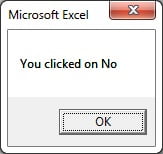
ELSEIF
Elseif is used to use more than one condition. See the below given example.
Sub IF_And_ElseIF() Dim x As Integer Dim y As Integer x = VBA.InputBox("Please enter the value to x") y = VBA.InputBox("Please enter the value to y") If x > y Then MsgBox "x is greater than y" ElseIf y > x Then MsgBox "y is greater than x" Else MsgBox "X is equal to Y" End If End Sub
When we will run this code, we must input x and y value as number.
I have given value of x as 5
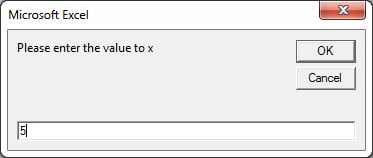
I have given value of y as 10
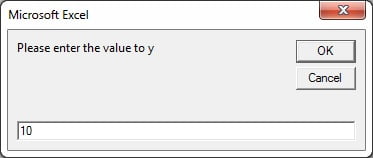
Now it will show the result
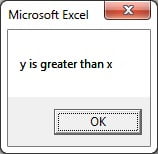
Watch the IF ELSE THEN tutorial video
Click here to download the practice file